Assignment 3: Interact#DES240
- Tingting F
- Oct 28, 2021
- 5 min read
DATA:Plastic pollution has the potential to cause the worst damage to seabirds in the seas around Aotearoa New Zealand, some 90 per cent of New Zealand's seabirds are threatened with extinction.
Team : <T+E+R>
Art Director : < Tingting >
Technical Lead : < Eric >
Data collector : <Ruby>

PROJECT PLAN
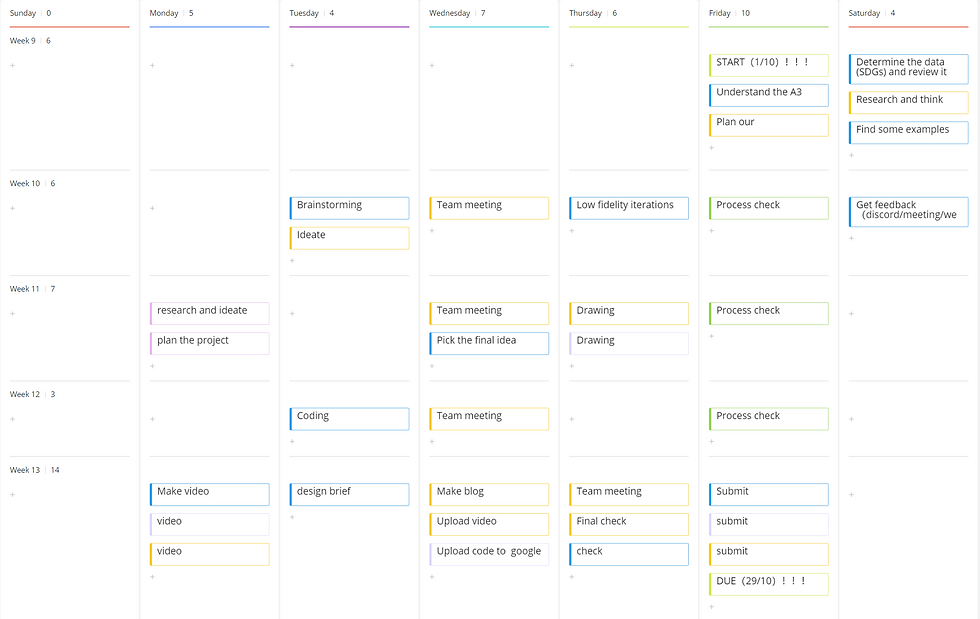
SKETCHES:
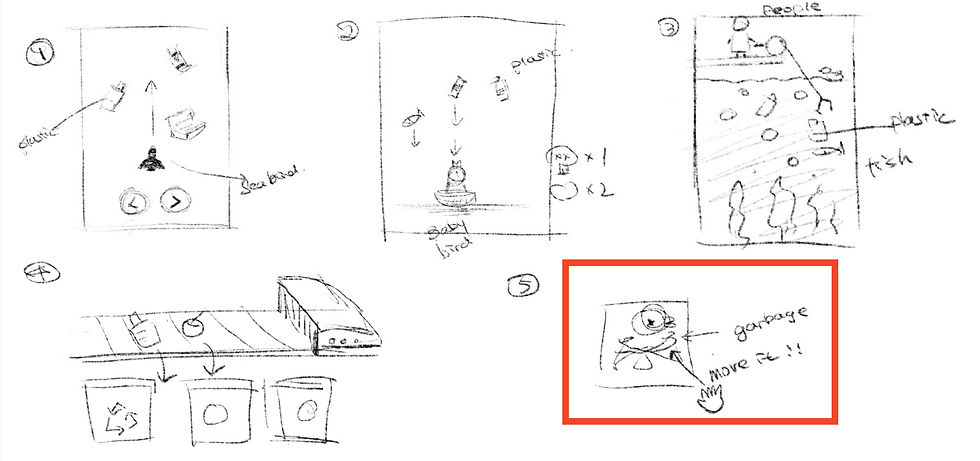
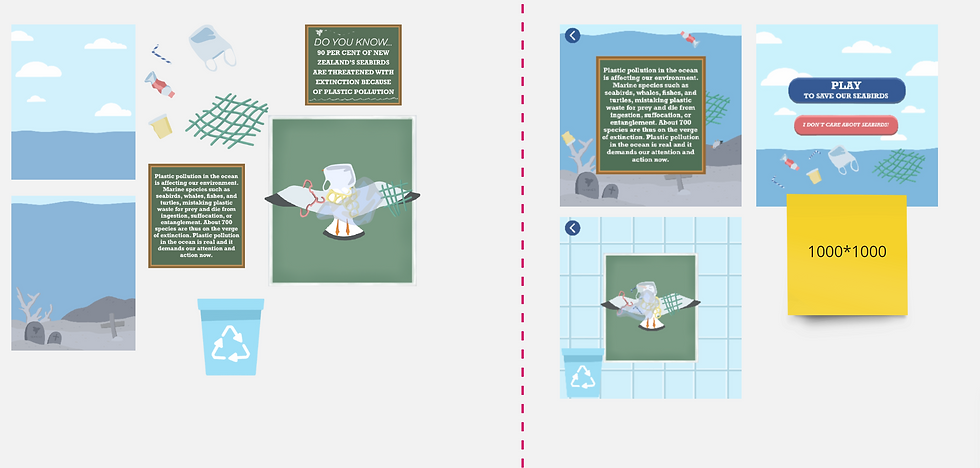
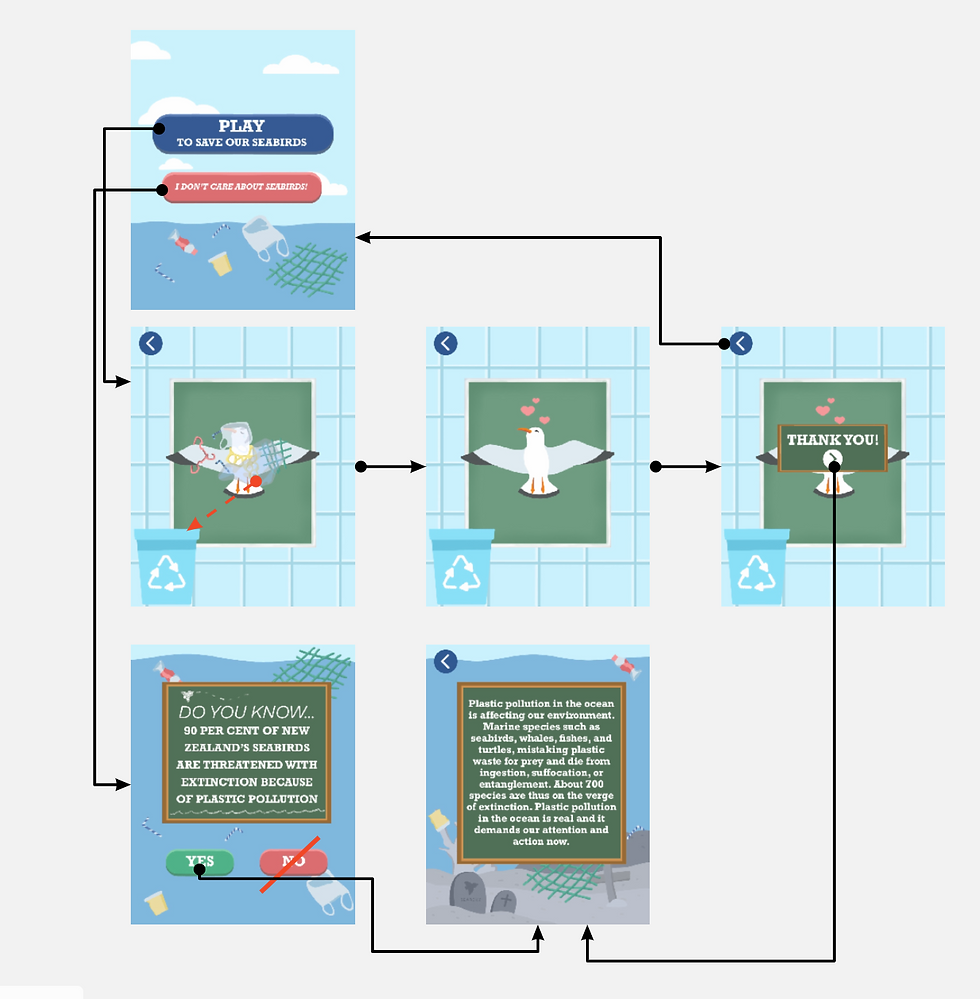
GIF:
Pitch:
CODE:
S1
class Scene1 extends Scene {
PImage[] plastics;
PVector[] locs;
float waveAmp = 8;
float waveFreq = 0.07;
Button b1, b2;
Scene1() {
back = loadImage("data/back/1.png");
plastics = new PImage[6];
locs = new PVector[6];
plastics[0] = loadImage("data/plastic/2.png");
plastics[1] = loadImage("data/plastic/7.png");
plastics[2] = loadImage("data/plastic/6.png");
plastics[3] = loadImage("data/plastic/5.png");
plastics[4] = loadImage("data/plastic/8.png");
plastics[5] = loadImage("data/plastic/9.png");
locs[0] = new PVector(110, 870);
locs[1] = new PVector(240, 750);
locs[2] = new PVector(410, 840);
locs[3] = new PVector(420, 710);
locs[4] = new PVector(600, 740);
locs[5] = new PVector(800, 850);
b1 = new Button(2, "1", "yes", width*0.5, 370);
b2 = new Button(3, "2", "red", width*0.5, 563);
}
void update() {
}
void render() {
pg.beginDraw();
pg.push();
pg.imageMode(CENTER);
pg.image(back, width *0.5, height *0.5);
for (int i=0; i<6; i++) {
wavePlastic(locs[i], plastics[i]);
}
b1.render(pg);
b2.render(pg);
pg.pop();
pg.endDraw();
}
void wavePlastic(PVector c, PImage p) {
float off = sin(c.x + frameCount*waveFreq) * waveAmp;
pg.image(p, c.x, c.y + off);
}
void onMousePressed() {
b1.onClick();
b2.onClick();
}
}
S2
class Scene2 extends Scene {
ArrayList<Plastic> plastics;
int dragId = -1;
PImage bird1, bird2;
PImage board;
PImage heart;
PGraphics bdPG;
PImage bin;
PVector binPos = new PVector(150, 850);
float binRange = 100;
int clearedCount = 0;
boolean allCleared = false;
int addHeartCD = 20;
int maxHeartNum = 4;
int heartNum = 0;
boolean addHeartDone = false;
Button rBtn;
MoveButton tkBtn;
Scene2() {
bin = loadImage("data/other/1.png");
heart = loadImage("data/other/2.png");
back = loadImage("data/back/2.png");
board = loadImage("data/back/3.png");
bird1 = loadImage("data/bird/1.png");
bird2 = loadImage("data/bird/2.png");
rBtn = new Button(1, "5", 75, 75);
tkBtn = new MoveButton(4, "3", width*0.5, height*0.5);
plastics = new ArrayList<Plastic>();
bdPG = createGraphics(board.width, board.height);
reset();
}
void update() {
if (!allCleared) {
AM.playBgm();
for (Plastic p : plastics) {
p.update();
if (dist(p.x, p.y, binPos.x, binPos.y) < binRange) {
if (!p.bCleared) {
p.bCleared = true;
clearedCount++;
AM.trigger("move");
println("cleared " + clearedCount);
if (clearedCount == plastics.size()) allCleared = true;
}
}
}
} else {
AM.stopBgm();
if (frameCount % addHeartCD == 0 && heartNum < maxHeartNum) {
addHearts(bdPG);
}
}
if (addHeartDone) tkBtn.move();
}
void render() {
pg.beginDraw();
pg.push();
pg.imageMode(CENTER);
pg.image(back, width *0.5, height *0.5);
pg.image(bdPG, width *0.5, height *0.5);
if (allCleared) {
pg.image(bird2, width *0.5, height *0.5);
} else {
pg.image(bird1, width *0.5, height *0.5);
for (Plastic p : plastics) {
p.render(pg);
}
}
pg.image(bin, binPos.x, binPos.y);
if (addHeartDone) tkBtn.render(pg);
rBtn.render(pg);
pg.pop();
pg.endDraw();
}
void addHearts(PGraphics pg) {
pg.beginDraw();
pg.imageMode(CENTER);
float scl = random(0.3, 0.9);
float x = random(210, 290);
float y = map(heartNum, maxHeartNum, 0, 20, 150);
pg.image(heart, x, y, heart.width*scl, heart.height*scl);
heartNum++;
if (heartNum == maxHeartNum) addHeartDone = true;
pg.endDraw();
}
void reset() {
plastics.clear();
plastics.add(new Plastic("10", 351, 472));
plastics.add(new Plastic("11", 494, 480));
plastics.add(new Plastic("12", 609, 462));
plastics.add(new Plastic("13", 473, 391));
plastics.add(new Plastic("14", 400, 382));
plastics.add(new Plastic("17", 508, 495));
plastics.add(new Plastic("18", 423, 502));
plastics.add(new Plastic("19", 536, 494));
clearedCount = 0;
allCleared = false;
heartNum = 0;
addHeartDone = false;
bdPG.beginDraw();
bdPG.imageMode(CORNER);
bdPG.image(board, 0, 0);
bdPG.endDraw();
dragId = -1;
for (Plastic p : plastics) {
p.bCleared = false;
}
tkBtn.reset();
}
void onMousePressed() {
for (int i = 0; i < plastics.size(); i++) {
Plastic d = plastics.get(i);
if (d.bHover) {
d.setClickOffset();
if ( dragId == -1 ) {
dragId = i;
}
break;
}
}
if (addHeartDone) tkBtn.onClick();
rBtn.onClick();
}
void onMouseDragged() {
if ( dragId == -1 ) return;
Plastic d = plastics.get( dragId );
d.setPos(mouseX, mouseY);
}
void onMouseReleased() {
dragId = -1;
}
}
S3
class Scene3 extends Scene {
PImage[] plastics;
PVector[] locs;
PImage board;
float waveAmp = 8;
float waveFreq = 0.07;
Button yesBtn;
Scene3() {
back = loadImage("data/back/4.jpg");
plastics = new PImage[6];
locs = new PVector[6];
board = loadImage("data/other/3.png");
plastics[0] = loadImage("data/plastic/2.png");
plastics[1] = loadImage("data/plastic/5.png");
plastics[2] = loadImage("data/plastic/6.png");
plastics[3] = loadImage("data/plastic/7.png");
plastics[4] = loadImage("data/plastic/8.png");
plastics[5] = loadImage("data/plastic/9.png");
locs[0] = new PVector(135, 655);
locs[1] = new PVector(470, 670);
locs[2] = new PVector(156, 922);
locs[3] = new PVector(244, 115);
locs[4] = new PVector(824, 875);
locs[5] = new PVector(785, 128);
yesBtn = new Button(4, "4", "yes", width*0.5, 773);
}
void update() {
}
void render() {
pg.beginDraw();
pg.push();
pg.imageMode(CENTER);
pg.image(back, width *0.5, height *0.5, width, height);
for (int i=0; i<6; i++) {
wavePlastic(locs[i], plastics[i]);
}
pg.image(board, width *0.5, 400);
yesBtn.render(pg);
pg.pop();
pg.endDraw();
}
void wavePlastic(PVector c, PImage p) {
float off = sin(c.x + frameCount*waveFreq) * waveAmp;
pg.image(p, c.x, c.y + off);
}
void onMousePressed() {
yesBtn.onClick();
}
}
S4
class Scene4 extends Scene {
PImage[] plastics;
PVector[] locs;
PImage board;
float waveAmp = 8;
float waveFreq = 0.07;
Button rBtn;
Scene4() {
back = loadImage("data/back/5.png");
plastics = new PImage[4];
locs = new PVector[4];
board = loadImage("data/other/4.png");
plastics[0] = loadImage("data/plastic/5.png");
plastics[1] = loadImage("data/plastic/6.png");
plastics[2] = loadImage("data/plastic/7.png");
plastics[3] = loadImage("data/plastic/9.png");
locs[0] = new PVector(890, 670);
locs[1] = new PVector(114, 625);
locs[2] = new PVector(870, 74);
locs[3] = new PVector(588, 817);
rBtn = new Button(1, "5", 75, 75);
}
void update() {
}
void render() {
pg.beginDraw();
pg.push();
pg.imageMode(CENTER);
pg.image(back, width *0.5, height *0.5, width, height);
for (int i=0; i<4; i++) {
wavePlastic(locs[i], plastics[i]);
}
pg.image(board, width *0.5, 450);
rBtn.render(pg);
pg.pop();
pg.endDraw();
}
void wavePlastic(PVector c, PImage p) {
float off = sin(c.x + frameCount*waveFreq) * waveAmp;
pg.image(p, c.x, c.y + off);
}
void onMousePressed() {
rBtn.onClick();
}
}
Plastic
final boolean DEBUG = false;
class Plastic {
float x, y;
float w, h;
PImage img;
boolean bHover;
boolean bCleared;
float xOff, yOff;
Plastic(String name, float x, float y) {
img = loadImage("data/plastic/" + name + ".png");
this.x = x;
this.y = y;
w = img.width;
h = img.height;
}
void update() {
bHover = false;
if (bCleared) return;
if ( mouseX > x - w/2 && mouseX < x + w/2 && mouseY > y - h/2 && mouseY < y + h/2 ) {
bHover = true;
}
}
void render(PGraphics pg) {
if (bCleared) return;
pg.imageMode(CENTER);
pg.image(img, x, y);
if (bHover && DEBUG) {
highLight(pg);
}
}
void setClickOffset() {
this.xOff = mouseX - x;
this.yOff = mouseY - y;
}
void setPos(float x, float y) {
this.x = x - xOff;
this.y = y - yOff;
}
void highLight(PGraphics pg) {
pg.push();
pg.rectMode(CENTER);
pg.noStroke();
pg.fill(255, 0, 0, 50);
pg.rect(x, y, w, h);
pg.pop();
}
}
ScenneManger
class SceneManager {
Scene activeScene;
Scene scene1, scene2, scene3, scene4;
SceneManager() {
scene1 = new Scene1();
scene2 = new Scene2();
scene3 = new Scene3();
scene4 = new Scene4();
LoadScene( 1 );
}
void Update() {
activeScene.update();
}
void Render() {
activeScene.render();
image(activeScene.pg, 0, 0);
}
void LoadScene(int index) {
println( "loadscene: ", index );
AM.stopBgm();
if (index == 1) activeScene = scene1;
else if (index == 2) {
activeScene = scene2;
activeScene.reset();
} else if (index == 3) activeScene = scene3;
else if (index == 4) activeScene = scene4;
}
void onMousePressed() {
activeScene.onMousePressed();
}
void onMouseDragged() {
activeScene.onMouseDragged();
}
void onMouseReleased() {
activeScene.onMouseReleased();
}
}
Scene
class Scene {
PGraphics pg;
PImage back;
int index;
Scene() {
pg = createGraphics(width, height);
}
void reset() {
}
void update() {
}
void render() {
pg.beginDraw();
pg.clear();
pg.endDraw();
}
void render(PGraphics pg) {
}
void onMousePressed() {
}
void onMouseDragged() {
}
void onMouseReleased() {
}
}
Commentaires